Understanding REST API: A Beginner's Guide
What is a REST Api?:
REST API, which stands for Representational State Transfer Application Programming Interface, is a set of rules and conventions for building and interacting with web services. RESTful APIs are designed to be simple, scalable, and stateless, making them widely used for various applications, including web and mobile development.
Here's an overview of REST API:
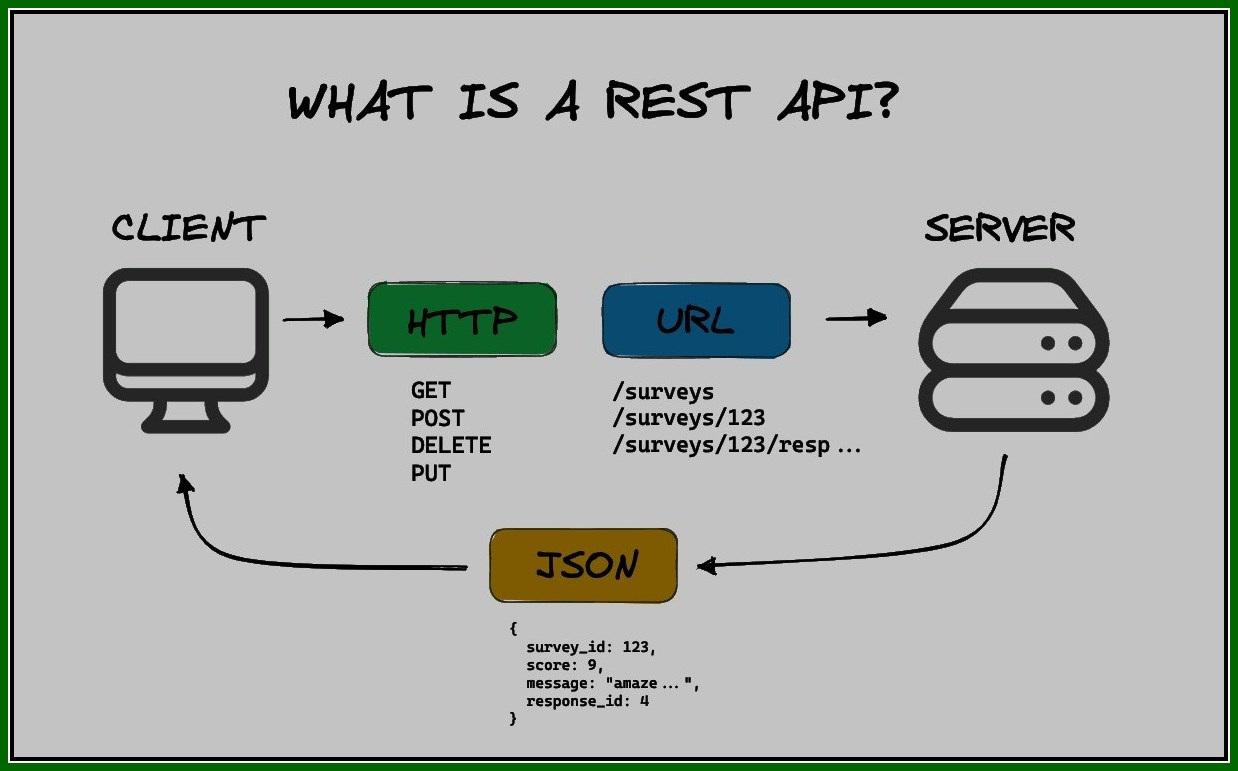
Why should we use a REST API?:
Using REST (Representational State Transfer) API has several advantages that make it a popular choice over other alternatives. Here are some reasons why REST API is commonly preferred:
Simplicity and Ease of Use: REST is designed to be simple and easy to understand. It uses standard HTTP methods (GET, POST, PUT, DELETE) and relies on a stateless communication model, making it intuitive for developers to work with.
Scalability: RESTful services are scalable because they are stateless. Each request from a client to a server contains all the information needed to understand and process the request. This allows servers to handle a large number of clients simultaneously.
Flexibility and Compatibility: REST is platform-independent and language-agnostic, making it compatible with a wide range of programming languages and frameworks. This flexibility enables interoperability between different systems.
Caching: REST supports caching mechanisms, allowing clients to cache responses. This can lead to improved performance and reduced server load, especially for frequently requested resources.
Wide Adoption: REST has become the de facto standard for designing APIs on the web. This widespread adoption means that there is a wealth of tools, libraries, and frameworks available to support the development and consumption of RESTful APIs.
Key Concepts of REST API:
Resources:
In a RESTful API, everything is treated as a resource. Resources are identified by URIs (Uniform Resource Identifiers).
HTTP Methods:
RESTful APIs use standard HTTP methods to perform operations on resources:
GET: Fetch information from a place.
POST: Add something new.
PUT: Change what's already there or add if it's not.
PATCH: Make a small update to what's already there.
DELETE: Remove something.
Uniform Interface:
The "Uniform Interface" feature in REST API ensures a consistent and standardized way for different systems to communicate. This common set of rules makes it simpler for them to exchange information, acting like a shared language that ensures reliable and predictable interactions.
Stateless:
Each request from a client to a server must contain all the information needed to understand and process the request. The server should not store any client state between requests.
Example:
Imagine you're ordering food at a restaurant (client to server interaction).
In a stateless system:
Each time you order, you have to specify your entire order.
The kitchen (server) doesn't remember your previous orders; you need to mention everything again.
The restaurant doesn't store information about your preferences or past orders.
So, in a stateless system, every interaction is independent, and the server doesn't retain any information about the client between requests.
Representation:
Resources can have different representations (e.g., JSON, XML). Clients can specify the desired representation using content negotiation.
Components of a REST API:
1. Endpoint (URI):
An endpoint is a specific URL or URI that represents a resource. It's the entry point for an API.
2. HTTP Methods:
Each endpoint supports one or more HTTP methods to perform specific actions on resources. For example:
GET
POST
PETCH
PUT
DELETE
3. Request:
Clients send HTTP requests to interact with the API. Requests include the HTTP method, headers, and optional data.
4. Response:
Servers respond to requests with an HTTP status code, headers, and the requested data in the desired representation format.
5. Status Codes:
HTTP status codes indicate the result of the request for Example:
200 OK
400 Bad Request
401 Unauthenticate
403 Forbidden
404 Not Found
500 Internal Server Error
REST API Installation Steps:
1. Design the API:
Plan the structure of your API, including the resources, endpoints, and the actions that can be performed.
2. Choose a Technology Stack:
Decide on the programming language and framework for building your API. Common choices include Node.js with Express, Django, Flask, Ruby on Rails, etc.
3. Implement Endpoints and Actions:
Write code to implement the defined endpoints and the corresponding actions using the chosen framework.
4. Test the API:
Use tools like Postman or cURL to test your API endpoints and ensure they behave as expected.
5. Document the API:
Provide documentation for your API, including details on available endpoints, expected request and response formats, and any authentication mechanisms.
6. Deploy the API:
Deploy your API to a server or a cloud platform to make it accessible to clients.
REST APIs are widely used for building web and mobile applications due to their simplicity, scalability, and ease of integration. They are a fundamental part of modern web development, enabling communication and data exchange between different systems.
While REST has these advantages, it's essential to consider the specific requirements of your project before choosing an API architecture. Other alternatives, such as GraphQL or SOAP, may be more suitable in certain situations based on factors like data complexity, real-time requirements, or existing infrastructure.